The Journey Begins: Functional Programming
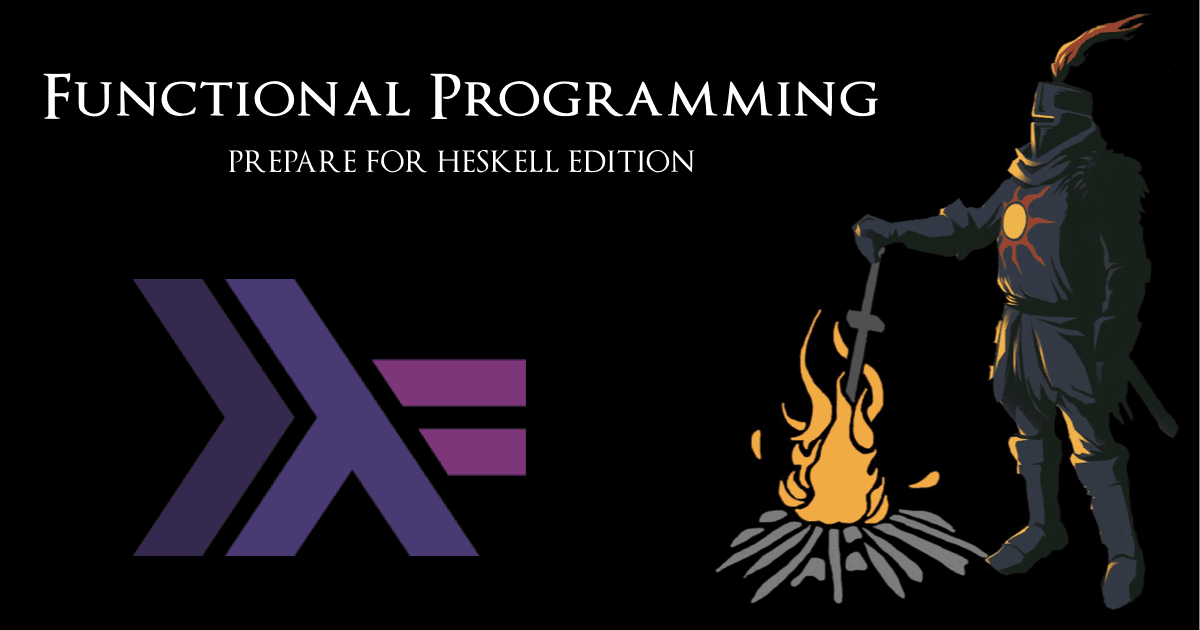
For a while now I wanted to dive into Functional Programming to properly learn how to use functional-lite concepts in JavaScript. It took me some time to window shop for a language that I was satisfied with. The main criteria for me were language usage, popularity, ease of tooling and, most important, learning material. I didn't want to learn just the language, my goal was to learn functional programming and the language should be the medium.
I've looked at Haskell and Elm. Considered Scala, Clojure, and F#. Checked out Racket, Lisp, and Scheme. And wondered about OCaml / ReasonML and Erlang. That's quite a list, and that's only scratching the surface.
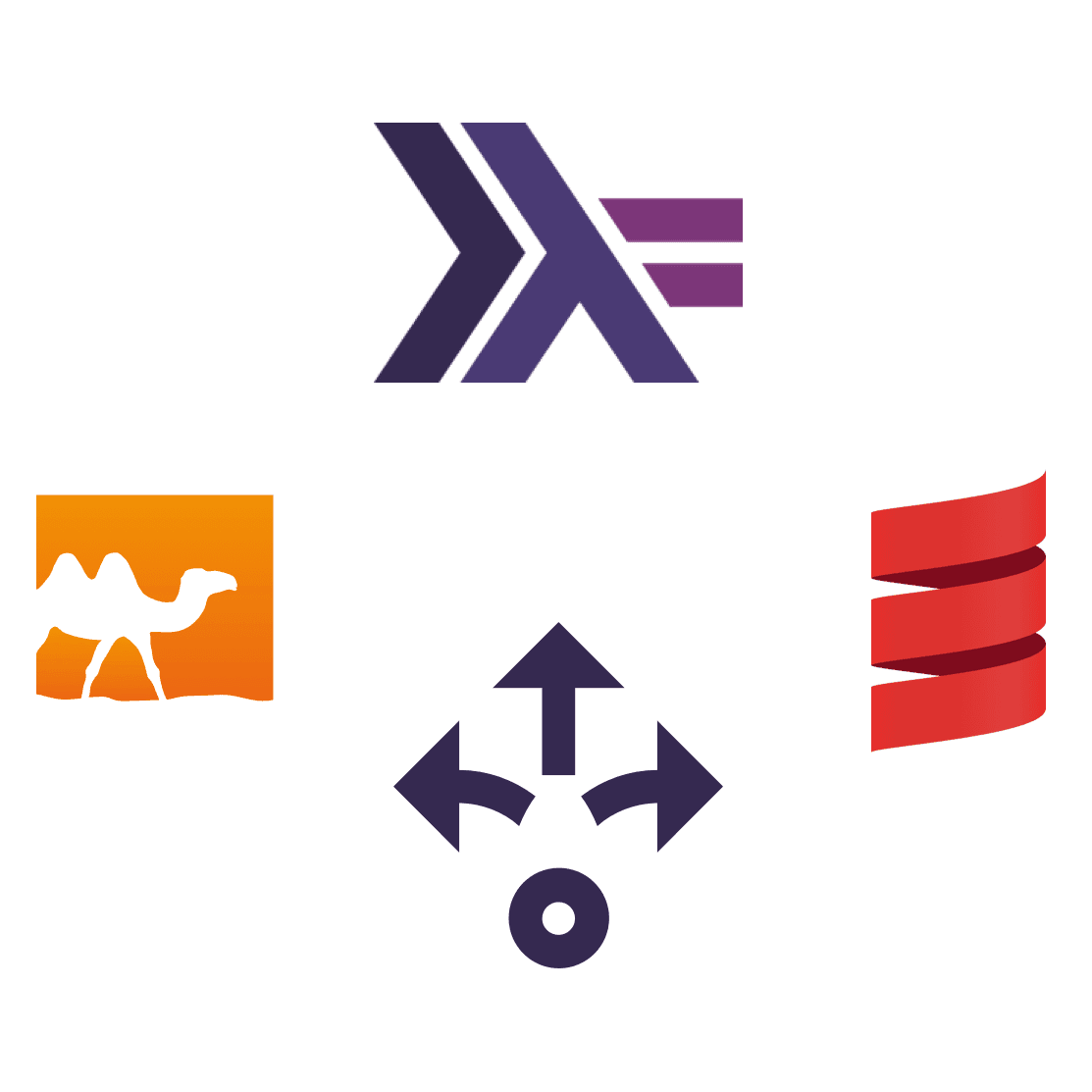
In the end, my choice fell on Haskell because of available learning materials and its purity. It would force me to learn how to properly use functional programming concepts and not revert back to imperative style while facing difficulties.
Companions
- Introduction to Functional Programming - The main purpose of this course is to introduce a complete beginner to functional programming with help of Haskell. The language is not the main focus, the course is free and in video format, just what I've been looking for.
- Learn You a Haskell - A free book that was made with a beginner to functional programming in mind.
- Real World Haskell - Another free book that is good for beginners and intermediate Haskell enthusiasts.
- Mostly Adequate Guide to FP - This is an excellent and free book that helps with learning how to apply functional programming concepts using JavaScript for daily usage.
- Programming in Haskell - I've heard good things about this book. Which is recommended by Erik Meijer, author of Introduction to Functional Programming course.
- Haskell Programming from first principles - This book was recommended a lot during my online search. A potential buy.
A Taste of Haskell
An quick example from Introduction to Functional Programming course to whet one's appetite for what's to come.
-- Quicksort
f :: (Ord a) => [a] -> [a]
f [] = []
f (x : xs) = ys ++ [x] ++ zs
where
ys = f [ a | a <- xs, a <= x ]
zs = f [ a | a <- xs, a > x ]
Beautiful.